In today’s digital era, web performance is crucial. Users demand fast, efficient online experiences, and slow websites can lead to frustration, higher bounce rates, and decreased conversions. As competition intensifies, optimising web performance becomes a strategic necessity for web developers to ensure user satisfaction and stay competitive in the dynamic online landscape.
Despite the critical importance of web performance, there’s a notable bias towards back-end optimization in the development landscape. Many developers and online resources prioritise server response times and database efficiency, often overlooking the impact of front-end performance. Neglecting aspects like file sizes can result in suboptimal user experiences, highlighting the need for a more balanced approach that addresses both back-end and front-end optimisations for a truly seamless digital presence.
There are several things we can do to optimise a website for front-end performance and some can even be done automatically. An easy first step could be to leverage a CDN, ensuring that our static assets such as images, stylesheets and JavaScript will be delivered faster. Using our own Accelerated Domains for example would improve the delivery of static content, optimise them and even optimise the images, reducing the file size significantly.
A second step could be to eliminate unneeded CSS and JavaScript code from being loaded. By dequeueing unused assets you can easily gain a notable performance boost on the front-end of your site. Some of the easiest pieces to eliminate are the ones that are loaded but that are not in use by your site.
How can we Identify What Files are Loaded That are not in use?
To identify unused assets on your website, we can use the built-in tools we already have in our browser. Most modern browsers have a “Coverage” tool in their built-in Developer Tools. We can leverage this to see what parts of our site are loaded while they are not in use. To open this in Chrome start by opening the Developer Tools. Then use CTRL-SHIFT-P on Windows and Linux, or Command-SHIFT-P on Mac to open the command menu. Start typing the word “coverage” and select Show Coverage from the menu. This will get you an additional window that looks like this:
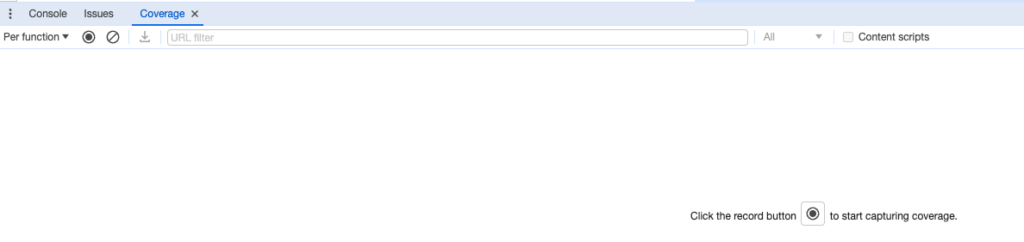
When opening this tool, the Coverage section is usually still blank. Getting a first overview is simple, just follow the instructions: click the record button, and reload the page you’re auditing. It should fill up looking like this:

The files we don’t need are easy to spot. They are the ones listed with 100% unused bytes in the column labelled as such.
Knowing What we Don’t use: Dequeue it!
So, we now know what files are loaded that are unneeded on this page. If these are loaded “the WordPress way” it’s fairly easy to prevent that from happening. For this, we rely on two functions: wp_dequeue_style() and wp_deregister_style(). And for us to use these functions we need to know the handles that are used to queue and register the styles initially. We could of course dig through the source code of our site, hoping to find the right handles for them. But, it’s easier to get those handles from the HTML source code generated by our site. When our stylesheet gets loaded, it will also be given an “id”, like this:
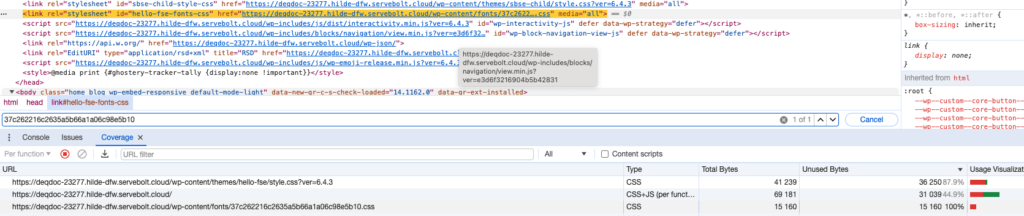
This id is the handle used to register and enqueue the file with WordPress, followed by the type of asset loaded. So -css
is added for stylesheets and -js
for JavaScript files. In our case, we can see the id used being hello-fse-fonts-css
. This tells us the handle we need to use is hello-fse-fonts
and the asset loaded is a stylesheet. All we need to do is reverse this process in our theme.
Note: if you haven’t created the theme but are relying on an external theme, ie: one from wordpress.org or an external theme shop, don’t edit the theme directly. Create a child theme, and make the changes there instead.
We can add the following code to our functions.php
file:
add_action( 'wp_enqueue_scripts', 'remove_unneeded_stylesheet', 20 );
function remove_unneeded_stylesheet() {
wp_dequeue_style( 'hello-fse-fonts' );
wp_deregister_style( 'hello-fse-fonts' );
}
We use add_action() to hook into the WordPress flow, at the point where scripts and stylesheets are normally loaded. The action we add is our function that deregisters and dequeues our scripts and stylesheets. In this example, we’ve only excluded one, but you can always add more. Save your changes and purge the cache for the page you’re testing and the stylesheet or JavaScript should now be gone. Freeing up network resources, and making it easier and quicker for the visitor to render your site.
But.. I Need Those Scripts on Another Page!
The above method is a bit blunt though as it will remove these scripts from every page on your WordPress site. Luckily, we can fix that. It’s just a matter of being a bit more specific when we are running our function. Instead of always dequeuing and deregistering, we can add exceptions for specific pages, post IDs, etc. All we need is to add some conditional logic like this:
if ( ! is_page( 42 ) ) {
wp_dequeue_style( 'hello-fse-fonts' );
wp_deregister_style( 'hello-fse-fonts' );
}
In this example, if the page doesn’t have the page ID 42, it will dequeue and deregister our stylesheet. If it is this page, however, they will still be loaded.
To target specific pages or posts, you can use functions like is_page() and is_single(). These can verify if the page we’re rendering is one of the ones we want or need. But don’t limit yourself to these. The WordPress developer documentation offers a wide variety of conditional checks you can make.
Conclusion
We’ve now tackled one front end hurdle for our site: the unneeded loading of files by the site. This way the front-end performance of our website should be increasing already. And this is just where we are getting started.
Keep in mind that for this strategy to be successful it’s important to map out the usage of these CSS and JavaScript files over the entire site. That way, you can tailor the solution to your exact needs.